Introduction to Auto Layout
Starting with iOS 6 Apple has given developers a powerful new way to create user interfaces that intelligently adapt to layout changes. This SDK addition, known as Auto Layout, enables a developer to express spatial relationships between views, leaving UIKit to update each view’s frame as needed to honor them. Relationships are expressed via layout constraints, which are objects of type NSLayoutConstraint, that can be added to a view by passing the addConstraint: or addConstraints: messages to it.
Auto Layout replaces the “springs and struts” autosizing layout mechanism used in the past. All new XIB and storyboard files have Auto Layout enabled by default. It is possible to port old views to use Auto Layout because it is smart enough to translate autosizing information into layout constraints.
The fact that Auto Layout was released at the same time as iPhone 5 is no coincidence. With iPhone 5’s screen being 4 inches tall, instead of the traditional 3.5 inches, there is a more pressing need to create adaptable user interfaces. If the rumored mini iPad is ever released, this concern will be even more pervasive for iOS developers. However, for simple views the old autosizing model works fine. For example, an app I have been developing at work relies heavily on UITableView and was created using autosizing, for running on iOS 5 and iPhone 4. When we tested the app out on iOS 6 and iPhone 5 simulator, there were almost no UI layout problems. For more complicated and custom user interfaces, however, the outcome probably won’t be as pain-free.
Auto Layout is also very helpful for supporting internationalization. Not only does it make the work of accommodating variable length display text easier, it also intrinsically supports right-to-left languages, such as Hebrew and Arabic. In the Auto Layout API the terms “leading” and “trailing” are used to generically refer to the start and end of a line, respectively. For left-to-right languages, such as English, leading means left and trailing means right. For right-to-left languages, it’s reversed. By relying on these generic concepts built into the API, it frees you, the application developer, from needing to take such locale-specific issues into account when building views.
How to use Auto Layout
Interface Builder (IB) now has support for working with layout constraints in an iOS app. It allows you to create and configure layout constraints on views without having to write a line of code. The screenshot below shows how constraints are now part of the document outline, and how the selected constraint is highlighted with a white border in the visual designer.

There is an ASCII-art inspired language named Visual Format Language (VFL) that can be used to ideographically express relationships between views. VFL text is written in code files, not IB, and acts as a facade over the cumbersome underlying API. Similar to the layout constraints support in IB, this language supports a subset of the functionality available in the API.

Most of the time the support in IB and VFL will cover your needs. However, the full power of layout constraints is available for situations that need it. Code can be written that creates individual NSLayoutConstraint objects and specifies all parameters required to create a single constraint, including things that are not available in other formats, such as a multiplier that allows a constraint to operate on a percentage of a value. The example below demonstrates this.
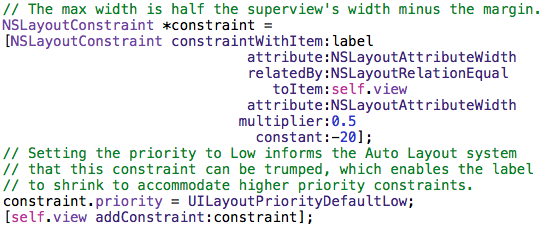
Cardinal rule of Auto Layout
There is something fundamental about Auto Layout that you must know in order to use it properly:
Constraints must provide enough information to determine one, and only one, size and location for a view.
If you don’t provide enough constraints, Auto Layout cannot accurately determine a view’s frame. If you provide too many constraints, such that multiple frames are possible for the same view, Auto Layout cannot accurately determine a view’s frame. If you violate the cardinal rule, either your app will crash or a large amount of helpful troubleshooting information will be logged to the Output window in Xcode.
Recommended Resources
There is far too much to say about Auto Layout for this introductory blog post. My goal here is to give a newcomer enough information about the topic so that it makes sense. If you have been working with iOS for a while, I’m sure you will see why it’s such an important topic to learn about. I suggest the following resources for an in-depth treatment of the subject:
The free tutorial on Ray Wenderlich’s site Beginning Auto Layout in iOS 6: Part 1/2
Both chapters about Auto Layout in Ray Wenderlich’s excellent book ‘iOS 6 by Tutorials’
Apple’s documentation: Cocoa Auto Layout Guide
All three presentations about Auto Layout at WWDC 2012
A simple demo project that shows how to work with Auto Layout in code onGitHub.