Using BLOB data type we can store an image in the SQLite database. The data that actually gets stored in the database is the bytes that make up an image or a file. The is the sixth tutorial in SQLite tutorial series and borrows its source code from the previous one. We will change the “DetailView” by adding a new section which will be used to display or change the image. The image can only be changed when the view is in the edit mode. We will use a UIImagePickerController to display the photo album and to select an image.
Changing the database
Let’s start by adding a new column to our “Coffee” database. Name the column “CoffeeImage” and set its data type to BLOB. This is how the database schema looks like inSQLite manager
Let’s start by adding a new column to our “Coffee” database. Name the column “CoffeeImage” and set its data type to BLOB. This is how the database schema looks like inSQLite manager
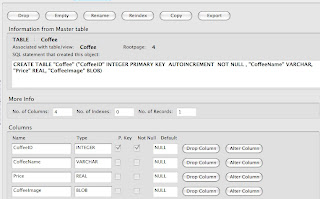
Changing the Coffee Class
Add a new property to the “Coffee” class which will hold the image from the database. This is how the header and the implementation files look like
The new property is synthesized and released in the dealloc method
Changing the Detail View
Let’s add a new row to the detail view, which will be used to display the image or to change the image in edit mode. Since the style of the table view placed on the detail view is set to “Grouped”, we will return three sections in numberOfSectionsInTableView and this is how the source code looks like
Let’s add a new row to the detail view, which will be used to display the image or to change the image in edit mode. Since the style of the table view placed on the detail view is set to “Grouped”, we will return three sections in numberOfSectionsInTableView and this is how the source code looks like
Let’s also display a title for the new section in tableView:titleForHeaderInSection. The code changes like this
Displaying the photo album
Now we need to display a photo album when the section is selected in edit mode. From this album we can select a image to be saved in the database. Using a UIImagePickerControllerwe can capture an image from the camera or the photo library on the device. UIImagePickerController class manages all the user interactions and all we have to do is display and dismiss it. Before we can display the view, we need to check if the interface is supported by calling isSourceTypeAvailable class method. The delegate of the UIImagePickerController should also confirm to UINavigationControllerDelegate andUIImagePickerControllerDelegate. In this case we will set the delegate of UIImagePickerController to “DetailViewController” and this is how the header and implementation file changes
Now we need to display a photo album when the section is selected in edit mode. From this album we can select a image to be saved in the database. Using a UIImagePickerControllerwe can capture an image from the camera or the photo library on the device. UIImagePickerController class manages all the user interactions and all we have to do is display and dismiss it. Before we can display the view, we need to check if the interface is supported by calling isSourceTypeAvailable class method. The delegate of the UIImagePickerController should also confirm to UINavigationControllerDelegate andUIImagePickerControllerDelegate. In this case we will set the delegate of UIImagePickerController to “DetailViewController” and this is how the header and implementation file changes
The variable “imagePickerView” is released in the dealloc method and the code listing is now shown here. We will initialize “imagePickerView” in viewDidLoad method and this is how the code changes
From the above code, “imagePickerView” is initialized and its delegate is set to self. This way “DetailViewController” will be notified of all the user actions on the picker controller. First, we need to display the view and it is done in tableView:didSelectRowAtIndexPath and this is how the code looks like
First confirm if the photo library is available on the device or not, if it is then present the view to the user. The method imagePickerController:didFinishPickingImage:editingInfo is called when an image is selected. This is how the code looks like
We get the selected image and set the “coffeeImage” property of the selected coffee. The table view is then reloaded and the picker view is dismissed.
Display the coffee image
We will display the coffee image in tableView:cellForRowAtIndexPath and this is how the code looks like
We will display the coffee image in tableView:cellForRowAtIndexPath and this is how the code looks like
If the index of the section is 2 then we set the title of the cell to “Change Image” and the image to the coffee image.
Saving image in the SQLite database
Until now we haven’t saved the image in the database because we only save data when the application is being terminated or if a memory warning is received. The data is saved in “saveAllData” method as seen in the previous tutorial. Let’s change this method to save the image in the database which now has a new column called “CoffeeImage” to hold the image as bytes. This is how the code looks like
Until now we haven’t saved the image in the database because we only save data when the application is being terminated or if a memory warning is received. The data is saved in “saveAllData” method as seen in the previous tutorial. Let’s change this method to save the image in the database which now has a new column called “CoffeeImage” to hold the image as bytes. This is how the code looks like
We first rewrite the update query to include the CoffeeImage column. We also need a way to get the image data as bytes and this is where UIImagePNGRepresentation method helps us. We then bind the BLOB parameter using sqlite3_bind_blob method. The first parameter takes the update statement, the second parameter takes the index of the parameter value, the third one is the actual data itself, the fourth one is the length of the data which is being saved, and a pointer to a method which is responsible to clean up the data. If sqlite3_bind_blob method does not return SQLITE_OK then we display an error in NSLog. If there is no error while saving the data then we have successfully saved the image in the SQLite database on the iPhone/iPod.
Getting an image from the SQLite database
We now have to get the data from the database when the detail view is loaded. This is done in “hydrateDetailViewData” method and this is how the code changes
We now have to get the data from the database when the detail view is loaded. This is done in “hydrateDetailViewData” method and this is how the code changes
The select query is changed to include CoffeeImage column and we usesqlite3_column_blob method to load the BLOB data into variable of type NSData. WE create an image of type NSData from imageWithData class method. The rest of the code works as described above.
This tutorial wasn’t designed to display imags anywhere and is a direct result of all the emails I got asking, how to save images in the SQLite database. So if you choose an image which does not fit in the section, it will take up all the space on the view.
Conclusion
This tutorial explains how to save and read images from a SQLite database. We can use the same functionality to save files in the database. I hope you had fun and learnt something new with this tutorial.
This tutorial explains how to save and read images from a SQLite database. We can use the same functionality to save files in the database. I hope you had fun and learnt something new with this tutorial.
Attachments
No comments:
Post a Comment